Sample - Monkey Job
The following is a sample Python code for calling QDC public APIs to test apk app.
- Available chipsets
Monkey jobs can only be used with job targets where the ChipsetCategory
is Mobile
. To get the list of available targets, call this QDC public API.
- Artifacts limitation
Type | Artifacts Number | Suffix |
---|---|---|
test package | required | .apk/.zip |
test script | N/A |
Install QDC Public API Python library
To get QDC Public API Python library, contact QDC support qdc.support@qti.qualcomm.com.
To install QDC Public API Python library, use the following command. Here, we use version 0.0.10 as an example:
pip install qdc_public_api_client-0.0.10-py3-none-any.whl
Reference for using Python library
For detailed instructions on each method in the Python library, please refer to Python Api Reference
A sample Python code to test an apk app
The following steps demonstrate the complete process of creating a new automated monkey job, including:
-
Preparing the parameters and submitting the job
-
Polling the job status
-
Polling the logs once the job is complete
-
Downloading all the logs
Step 1: Create a new python file
-
Create a file named hello_qdc.py
-
Copy and paste the following sample code into hello_qdc.py
import osimport timefrom qdc_public_api_client.models import JobType, JobMode, TestFramework, ArtifactTypeimport qdc_apiif __name__ == '__main__':api_key = "" # copy from user settingspublic_api_client = qdc_api.get_public_api_client_using_api_key(app_name_header="QDC User",client_type_header="appName",api_key_header=api_key)# Section (1): preparing the parameters and submitting the jobjob_device_target = "SM8650" # device target chipsettest_package_file = r"./MonkeyExample.apk" # same name as the apk preparedtest_package_response = qdc_api.upload_file(public_api_client, test_package_file, ArtifactType.TESTPACKAGE)job_target_id = qdc_api.get_target_id(public_api_client, job_device_target)job_id = qdc_api.submit_job(public_api_client=public_api_client,target_id=job_target_id,job_name="Example Job",external_job_id="ExJobId001",job_type=JobType.AUTOMATED,job_mode=JobMode.APPLICATION,timeout=600,test_framework=TestFramework.MONKEY,entry_script="",job_artifacts=[test_package_response],monkey_events=500,monkey_session_timeout=100,job_parameters=[])# Section (2): polling the job statuscompleted = Falsechecked = 0while not completed:poll_job_status = qdc_api.get_job_status(public_api_client, job_id)if poll_job_status.lower() == "completed":print("Job is completed, exiting client...")breakelif poll_job_status.lower() == "canceled":print("Job is canceled, exiting client...")breakelse:print(f"Job is in {poll_job_status.lower()} mode, waiting for 15 seconds.")time.sleep(15)checked = checked + 1if checked == 20: # After polling 20 times aborting the jobabort_job = qdc_api.abort_job(public_api_client, job_id) # To abort the submitted jobsprint(f"Job state {abort_job}")# Section (3): polling the logs once the job is completeget_job_log_files_response = []logs_checked = 0while not get_job_log_files_response:get_job_log_files_response = qdc_api.get_job_log_files(public_api_client, job_id)if not get_job_log_files_response:print(f"Job is completed and the server is uploading logs, waiting for 30 seconds.")time.sleep(30)logs_checked = logs_checked + 1if logs_checked == 20: # Exists after polling 20 timesprint(f"No log files found, exiting...")print(f"Job {job_id} logs: {get_job_log_files_response}")# Section (4): downloading all the logsif get_job_log_files_response:for job_log in get_job_log_files_response:target_path = f"./logs/{job_log.filename}.zip"target_dir = os.path.dirname(target_path)os.makedirs(target_dir, exist_ok=True)qdc_api.download_job_log_files(public_api_client, job_log.filename, target_path)
Step 2: Prepare test package
Prepare an apk file and move it into the same folder as hello_qdc.py. This apk file will be uploaded to remote device during the test.
Step 3: Execute and check the Logs
- Run the following command.
python hello_qdc.py
- After successful execution, the job logs will be saved in the current folder.
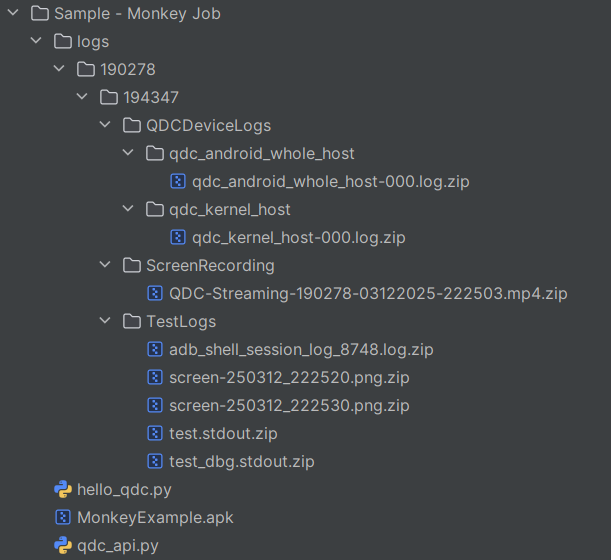